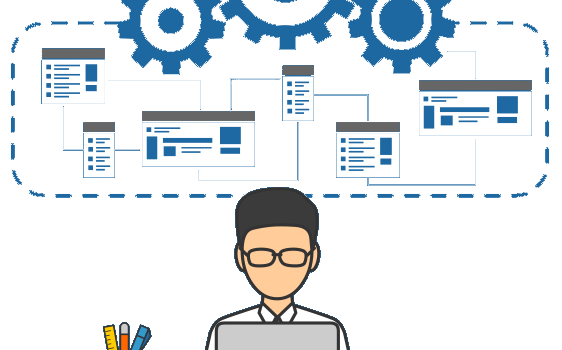
Selenium Assignment – 1
Here is the first assignment of this series of real-time scenarios, to practice Selenium from TestingBuddy. This assignment mainly focuses on how to invoke the Firefox browser, maximizing the window, navigate commands, etc.
This assignment is not considering proper framework development. Once you are good with frameworks, try this again considering framework.
Note: If you are very new to selenium, go through Environment setup for Selenium WebDriver and other articles before starting.
Scenario:
- Open the Firefox browser.
- Maximize the browser window.
- Navigate to “https://testingbuddy.co.in/?p=169”.
- Write a method to print PASS if the title of the page matches with “QA Automation Tools Trainings and Tutorials | QA Tech Hub” else FAIL. (If you are familiar with TestNG or JUnit use assert statement like assert.assertequals(actual, expected) to give a verdict of the pass or fail status.
- Navigate to the Facebook page (https://www.facebook.com)
- Navigate back to the QA Tech Hub website.
- Print the URL of the current page.
- Navigate forward.
- Reload the page.
- Close the Browser.
Try this code. Send us your feedback and if you have any questions, queries or comments.
package com.test.traveltest;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class Assignment1 {
FirefoxDriver driver;
String tbUrl = "https://testingbuddy.co.in/?p=169";
String facebookUrl = "https://www.facebook.com";
@BeforeTest
public void invokeBrowser(){
System.setProperty("webdriver.gecko.driver",
"D:\\Selenium\\geckodriver-v0.31.0-win64\\geckodriver.exe");
driver = new FirefoxDriver();
driver.manage().window().maximize();
driver.manage().timeouts().pageLoadTimeout(20, TimeUnit.SECONDS);
driver.get(tbUrl);
}
@Test(priority=0)
public void Test1() {
String titleOfThePage = driver.getTitle();
System.out.println(titleOfThePage);
Assert.assertEquals(titleOfThePage, "Difference between abstract class and interface - Testingbuddy");
}
@Test(priority= 1)
public void Test2() {
driver.navigate().to(facebookUrl);
String currentUrl = driver.getCurrentUrl();
System.out.println("Current URL :: " + currentUrl);
driver.navigate().back();
driver.navigate().forward();
driver.navigate().refresh();
}
@AfterTest
public void testComplete() {
driver.quit();
}
}
2 COMMENTS
Nice post. I learn something new and challenging on sites I stumbleupon everyday. Its always interesting to read content from other writers and practice a little something from their sites.
Thankyou