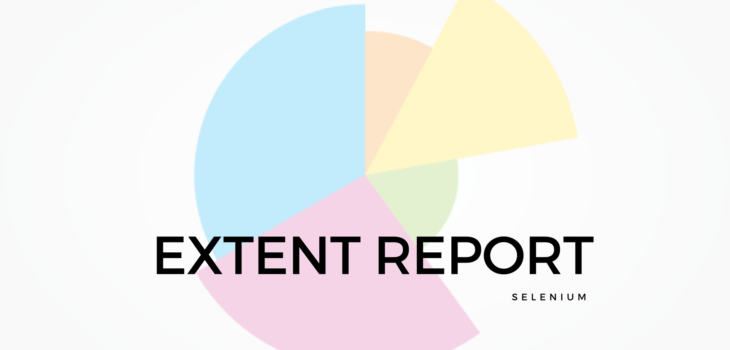
Generate Extent Reports in Selenium Webdriver
What are the Extent Reports?
ExtentReports is an open-source reporting library used in selenium test automation. Extent reports become the first choice of Selenium Automation Testers, even though Selenium comes with inbuilt reports using frameworks like JUnit and TestNG. With extent reports, you can offer a more extensive and insightful perspective on the execution of your automation scripts. So let’s see why Automation testers prefer Extent reports to others.
Advantages of Extent Reports
Some of the advantages of Extent Reports are as follows
- Extent reports are more customizable than others.
- Captures screenshots at every test step
- Displays the time taken for test case execution within the report.
- Extent API can produce more interactive reports, a dashboard view, graphical view, and emailable reports
- You can easily track multiple test case runs that occur within a single suite.
- It can be easily integrated with frameworks like JUnit, NUnit, & TestNG
Steps To Generate Extent Reports
Step #1: Firstly, create a TestNG project in eclipse
Step #2: Download extent library files (Extent reports Version 5 JAR file – Extent Spark Reporter) and add the downloaded library files to your project
If you are using pom.xml file then you can add Extent Reports Maven dependency 5.0.9 in your maven project.
<dependency>
<groupId>com.aventstack</groupId>
<artifactId>extentreports</artifactId>
<version>5.0.9</version>
</dependency>
Step #3: Create a java class say ‘ExtentReportsClass’ and add the following code to it
Code to validate title and logo on Google home page.
Given clear explanation in the comments section with in the program itself. Please go through it to understand the flow.
package com.test.traveltest;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.ITestResult;
import org.testng.SkipException;
import org.testng.annotations.*;
import com.aventstack.extentreports.ExtentReports;
import com.aventstack.extentreports.ExtentTest;
import com.aventstack.extentreports.Status;
import com.aventstack.extentreports.markuputils.ExtentColor;
import com.aventstack.extentreports.markuputils.MarkupHelper;
import com.aventstack.extentreports.reporter.ExtentSparkReporter;
//import com.aventstack.extentreports.reporter.configuration.ChartLocation;
import com.aventstack.extentreports.reporter.configuration.Theme;
public class ExtentReportsClass {
public WebDriver driver;
public ExtentSparkReporter spark;
public ExtentReports extent;
public ExtentTest logger;
@BeforeTest
public void startReport() {
// Create an object of Extent Reports
extent = new ExtentReports();
spark = new ExtentSparkReporter(System.getProperty("user.dir") + "/test-output/RKExtentReport.html");
extent.attachReporter(spark);
extent.setSystemInfo("Host Name", "Testing Buddy");
extent.setSystemInfo("Environment", "Production");
extent.setSystemInfo("User Name", "Rahulkundu");
spark.config().setDocumentTitle("Title of the Report Comes here ");
// Name of the report
spark.config().setReportName("Name of the Report Comes here ");
// Dark Theme
spark.config().setTheme(Theme.STANDARD);
}
//This method is to capture the screenshot and return the path of the screenshot.
public static String getScreenShot(WebDriver driver, String screenshotName) throws IOException {
String dateName = new SimpleDateFormat("yyyyMMddhhmmss").format(new Date());
TakesScreenshot ts = (TakesScreenshot) driver;
File source = ts.getScreenshotAs(OutputType.FILE);
// after execution, you could see a folder "FailedTestsScreenshots" under src folder
String destination = System.getProperty("user.dir") + "/Screenshots/" + screenshotName + dateName + ".png";
File finalDestination = new File(destination);
FileUtils.copyFile(source, finalDestination);
return destination;
}
@BeforeMethod
public void setup() {
System.setProperty("webdriver.chrome.driver",System.getProperty("user.dir")+"\\src\\main\\java\\drivers\\chromedriver.exe");
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.google.com/");
}
@Test
public void verifyTitle() {
logger = extent.createTest("To verify Google Title");
Assert.assertEquals(driver.getTitle(),"Google");
}
@Test
public void verifyLogo() {
logger = extent.createTest("To verify Google Logo");
boolean img = driver.findElement(By.xpath("//img[contains(@class,'lnXdpd')]")).isDisplayed();
logger.createNode("Image is Present");
Assert.assertTrue(img);
logger.createNode("Image is not Present");
Assert.assertFalse(img);
}
@Test
public void verifySearchEnabled() {
logger = extent.createTest("To verify search field");
boolean img1 = driver.findElement(By.xpath("//img[contains(@class,'lnXdpd')]")).isEnabled();
logger.createNode("Text field is enabled ");
Assert.assertTrue(img1);
}
@Test
public void verifySearch() {
logger = extent.createTest("To verify search works");
driver.findElement(By.xpath("//input[@name='q']")).sendKeys("rahul");
logger.createNode("rahul entered for search");
driver.findElement(By.className("gNO89b")).click();
logger.createNode("search button is clicked");
}
@AfterMethod
public void getResult(ITestResult result) throws Exception{
if(result.getStatus() == ITestResult.FAILURE){
//MarkupHelper is used to display the output in different colors
logger.log(Status.FAIL, MarkupHelper.createLabel(result.getName() + " - Test Case Failed", ExtentColor.RED));
logger.log(Status.FAIL, MarkupHelper.createLabel(result.getThrowable() + " - Test Case Failed", ExtentColor.RED));
//To capture screenshot path and store the path of the screenshot in the string "screenshotPath"
//We do pass the path captured by this method in to the extent reports using "logger.addScreenCapture" method.
//String Scrnshot=TakeScreenshot.captuerScreenshot(driver,"TestCaseFailed");
String screenshotPath = getScreenShot(driver, result.getName());
//To add it in the extent report
logger.fail("Test Case Failed Snapshot is below " + logger.addScreenCaptureFromPath(screenshotPath));
}
else if(result.getStatus() == ITestResult.SKIP){
logger.log(Status.SKIP, MarkupHelper.createLabel(result.getName() + " - Test Case Skipped", ExtentColor.ORANGE));
}
else if(result.getStatus() == ITestResult.SUCCESS)
{
logger.log(Status.PASS, MarkupHelper.createLabel(result.getName()+" Test Case PASSED", ExtentColor.GREEN));
}
driver.quit();
}
@AfterTest
public void endReport() {
extent.flush();
}
}