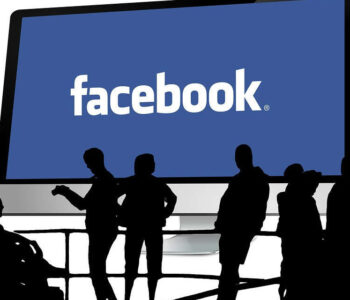
Selenium Assignment – 2
This is the second assignment in the series of real-time scenarios
Facebook Sign up:
Scenario:
- Open a Firefox browser.
- Navigate to “http://www.facebook.com/register”
- Verify that the page is redirected to “http://www.facebook.com/register”, by getting the current URL. use Assert.assertequals() in case you are familiar with TestNG or JUnit
- Verify that there is a “Create an account” section on the page.
- Fill in the text boxes: First Name, Surname, Mobile Number or email address, “Re-enter mobile number”, new password.
- Update the date of birth in the drop-down.
- Select gender.
- Click on “Create an account”.
- Verify that the account is created successfully.
package com.test.traveltest;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.Select;
import org.testng.Assert;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class Assignment2 {
WebDriver driver;
String fbUrl = "https://www.fb.com";
String facebookUrl = "https://www.facebook.com/register";
@BeforeClass
public void invokeBrowser(){
System.setProperty("webdriver.gecko.driver",
"D:\\Selenium\\geckodriver-v0.31.0-win64\\geckodriver.exe");
driver = new FirefoxDriver();
driver.manage().window().maximize();
driver.manage().timeouts().pageLoadTimeout(20, TimeUnit.SECONDS);
driver.get(facebookUrl);
}
@Test
public void verifyURL(){
String urlFromBrowser = driver.getCurrentUrl();
Assert.assertEquals(urlFromBrowser, facebookUrl, "No redirection happened");
}
@Test
public void facebookSignUp() {
driver.findElement(By.name("firstname")).sendKeys("Test");
driver.findElement(By.name("lastname")).sendKeys("User");
driver.findElement(By.name("reg_email__")).sendKeys("testuser@test.com");
driver.findElement(By.name("reg_passwd__")).sendKeys("testPassword");
Select selDate = new Select(driver.findElement(By.id("day")));
Select selMonth = new Select(driver.findElement(By.id("month")));
Select selYear = new Select(driver.findElement(By.id("year")));
selDate.selectByVisibleText("21");
selMonth.selectByVisibleText("Jun");
selYear.selectByVisibleText("1989");
driver.findElement(By.xpath("//input[@type='radio' and @value='2']")).click();
driver.findElement(By.xpath("//button[text()='Sign Up']")).click();
}
@AfterClass
public void closeBrowser(){
driver.quit();
}
}