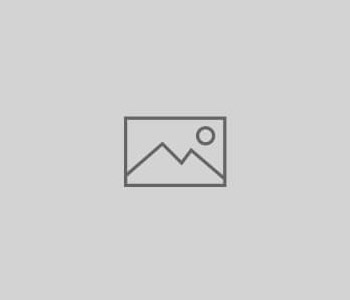
How to automate gmail login process using selenium webdriver…
We can automate the Gmail login process using Selenium webdriver in Java. To perform this task, first we have to launch the Gmail login page and locate the email, password and other elements with the findElement method and then perform actions on them.
Let us have the look at the Gmail login page −

Code Implementation
package RK1.Building_a_selenium_project;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
public class GmailLogin {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "F:\\Work Environment\\MyProject\\QA_Round\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
//implicit wait
driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS);
//URL launch
driver.get("https://accounts.google.com/signin");
//identify email
WebElement l = driver
.findElement(By.name("identifier"));
l.sendKeys("abc@gmail.com");
WebElement b = driver
.findElement(By.className("VfPpkd-LgbsSe"));
b.click();
//identify password
WebElement p = driver
.findElement(By.name("password"));
p.sendKeys("123456");
b.click();
//close browser
driver.close();
}
}
Leave a Reply